Getting Started With The cPanel® API
cPanel & WHM’s web interfaces offer a comprehensive server and web hosting management solution, but hosts and developers often want to automate tasks or integrate cPanel’s tools with other software. To make that easier, we provide extensive APIs that can be accessed both locally and remotely.
In this article, we’ll explore the cPanel & WHM APIs and show you how to get started with cPanel’s command-line API utilities and the HTTP API. We have recently updated our API documentation as well at https://api.docs.cpanel.net/
What Is The cPanel API?
The cPanel & WHM APIs (Application Programming Interfaces) allow users to access the software’s built-in functionality without going through the web interface. To use the APIs, you send a command, and cPanel responds by carrying out an action, which could be to change a setting, perform a server administration task, or tell you some information.
There are several benefits to using the APIs.
- They allow users to automate tasks rather than do them manually. For example, as we’ll see in the next section, you can trigger a backup from a script using the API. That script could run on the same server as cPanel or a different server that sends commands over the internet.
- They allow cPanel to integrate with other tools. A web host might use the API to collect data to display in a dashboard.
- You can use the APIs to extend cPanel’s functionality. Many cPanel extensions build on the APIs.
In short, our APIs help users and developers to leverage cPanel’s sophisticated server and hosting administration technologies to solve problems, save time, and build flexible tools for their users.
Accessing the cPanel API From Your Server
We provide a pair of command-line utilities for scripting and running commands on your server or hosting account, one for the WHM API (whmapi) and the cPanel API (uapi). Functionality is split between them in the same way it’s split between the WHM and cPanel web interfaces, so you would use whmapi for server-related tasks and uapi for hosting-related tasks.
To use them, you need to be logged in to your server with SSH.
First, let’s see how to query the WHM API for information about a server’s user accounts.
whmapi list_users
We’re using the “whmapi” command to call the “list_users” function. It does precisely what you’d expect, returning a list of users who have accounts on the server, along with some metadata about the command.
data: users: - root - user1 - user2 - example1 metadata: command: list_users reason: OK result: 1 version: 1
Now we know which users have accounts on the server, we want to take a deeper look at one account in particular.
whmapi1 accountsummary user=user1
Here we’re using the “accountsummary” function, and providing the parameter “user” with the value “user1”. A truncated set of results looks like this:
data: acct: - backup: 1 disklimit: unlimited diskused: 367M domain: example.com email: user1@example.com ip: 198.51.100.13
We see that the account has a domain associated with it, so let’s use the cPanel API to find more information.
One common unknown when starting with our API is understanding how masquerading works. Although the cPanel API is designed to be run as the cPanel user, a root user can masquerade as the cPanel user by specifying `–user=<username>` in the UAPI command.
uapi – user=user1 DomainInfo list_domains
The username is given as an option rather than a parameter. Many functions are gathered into modules that provide related functionality. In this case, we’re accessing the “DomainInfo” module and its “list_domains” function. It returns information about all the domains associated with the account.
data: addon_domains: - example3.com main_domain: example.com parked_domains: [] sub_domains: - files.example.com
So far we’ve only queried the APIs for information, but they can also be used to instruct cPanel to carry out tasks. For example, let’s say we want to trigger a full backup of this user’s account. To do that, we’d use the “Backup” module with the “fullbackup_to_homedir” function:
uapi – user=user1 Backup fullbackup_to_homedir
Each API includes many modules and dozens of functions capable of automating much of the functionality provided by cPanel & WHM. You will find a full list of modules and more information about using the command-line API utilities in the documentation.
Accessing the cPanel API Remotely
In addition to their command-line utilities, you can query both APIs remotely with HTTP requests. Any programming language or tool capable of sending HTTP requests can access API functions, but we’re going to use the “curl” command-line tool for our demonstration; to follow along make sure you have curl installed on your system. It’s available on Linux from your package manager, on macOS via brew, and on Windows via the Chocolatey package manager.
It wouldn’t be safe to allow just anyone to interact with cPanel’s API, so every request needs to be authenticated and linked to a user with the right permissions. There are various authentication mechanisms, but we’re going to use authentication tokens.
cPanel and WHM Authentication Tokens
An authentication token is a long string of letters and numbers that grants access to the API. Both WHM and cPanel include an interface for generating tokens called Manage API Tokens. They’re similar, so we’ll just walk you through the process of creating a token in WHM.
Navigate to the Manage API Tokens page, which you’ll find in the sidebar menu under Development. Click the Generate Token button, which opens a page for you to select the specific privileges you’d like to give the token holder.
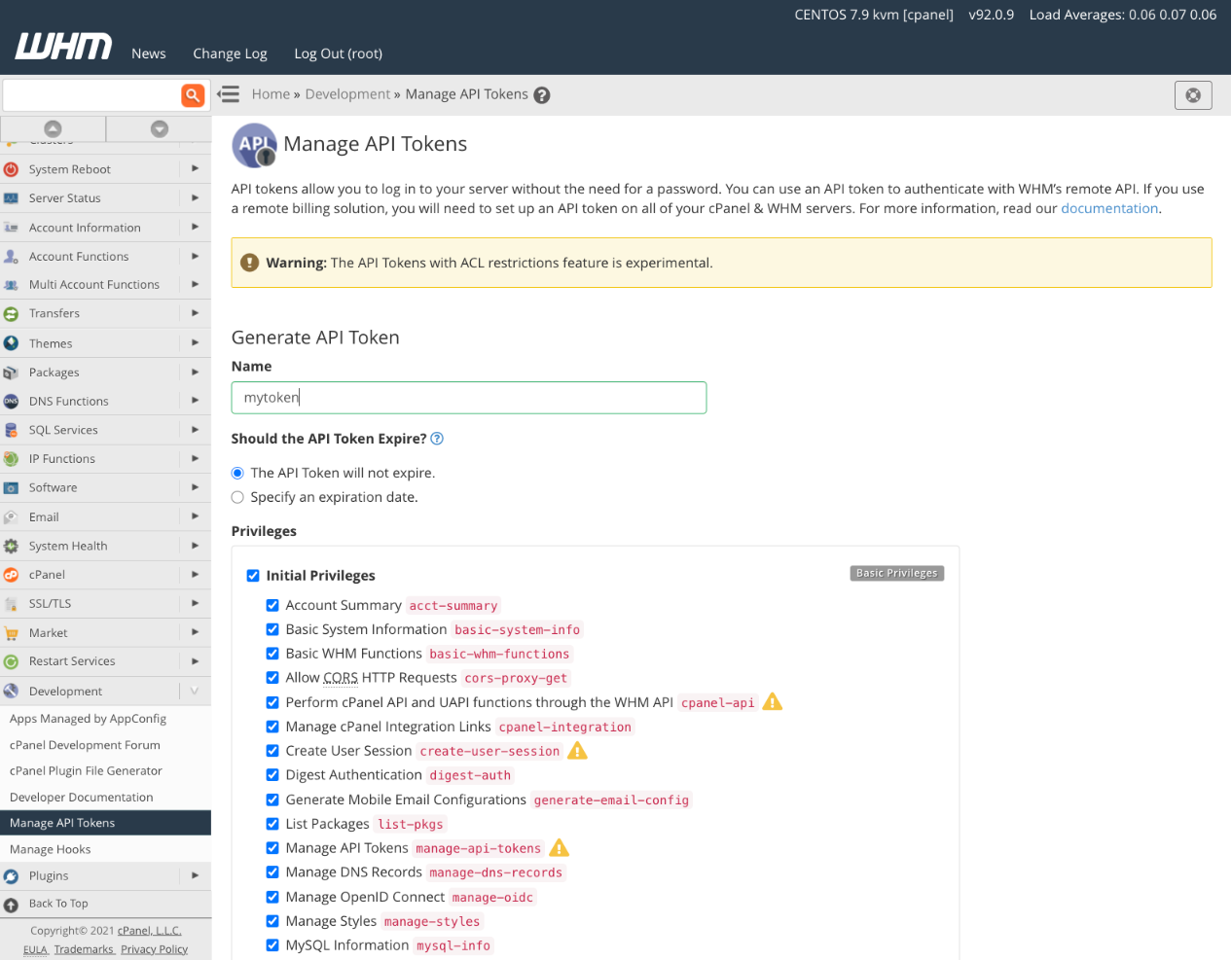
You should give authentication tokens only necessary privileges; if a bad actor gets their hands on one with a broad range of privileges, they may gain access to the entire system.
Give it a meaningful name and choose an optional expiration date before clicking Save at the bottom of the page. WHM generates the token and displays it for you to copy. Once you leave this page, you can’t access it again, so be sure to save it somewhere safe.
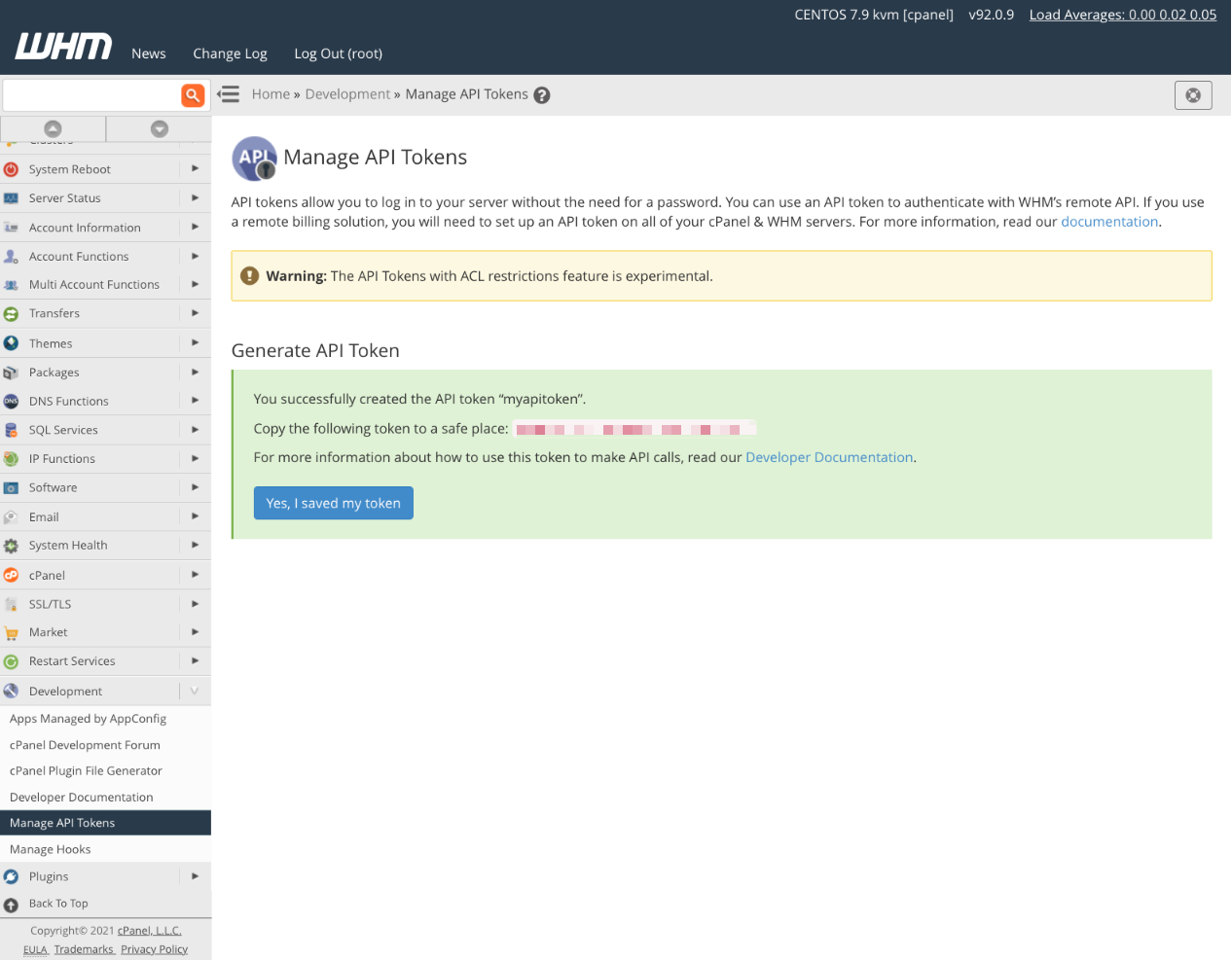
Making API Calls with Curl
We can remotely access all of the same API functions we previously used when logged in to the server. To try it out, we’ll list the server’s users as we did before with the command-line utility.
curl -H'Authorization: whm root:XXXXXXXXXXXXXXXXXXX' ‘https://example.com:2087/json-api/list_users?api.version=1’
This looks complicated, so let’s break it down:
- curl — the command-line HTTP client we’re using to send the request to our cPanel server.
- -H’Authorization: whm root:XXXXXXXXXXXXXXXXXXX’ — the -H option tells curl to send an HTTP header with authentication information; in this case, the API we’re accessing, the root user or the name of the reseller account associated with the token, and the token itself. You should replace XXXX with your token.
- https://example.com:2087/ — the domain and port of our server. The port will be different depending on the API we are contacting. For a secure SSL connection to WHM, we use 2087.
- json-api — JSON is a data format, and we’re telling the API to send JSON data in response to our request.
- list_users?api.version=1 — the API function to call and the API version we’re using.
You can find more information about the structure of API calls in the documentation.
When you send the request, curl prints out the JSON data it gets back from WHM.
{ "metadata" : { "command" : "list_users", "result" : 1, "reason" : "OK", "version" : 1 }, "data" : { "users" : [ "root", "user1", "user2", "example1" ] }
As you can see, it’s the same information we got from the command-line app in a more programmer-friendly format.
In our final example, we’ll use the cPanel API to retrieve domain information for user1, just as we did with the uapi app in the previous section.
curl -H'Authorization: cpanel user1:XXXXXXXXXXXXXX' 'https://example.com:2083/execute/DomainInfo/list_domains'
The format of the request is the same, but some details have changed. We’re using the secure cPanel port (2083), and the module (DomainInfo) and function (list_domains) are preceded by “execute”, which tells cPanel to expect a command. Additionally, we’re accessing the API using a token associated with a cPanel user, so we have to provide their username in the authentication header.
Each module and function has a page in the documentation. For example, the list_domains function. If you’re unsure which format to use for a function or which modules and functions are available, the documentation should be your first port of call. The cPanel & WHM APIs empower server administrators and hosting clients to take advantage of the software’s rich functionality to build their scripts, automations, and integrations. As always, if you have any feedback or comments, please let us know. We are here to help in the best ways we can. You’ll find us on Discord, the cPanel forums, and Reddit. Be sure to also follow us on Facebook, Instagram, and Twitter.